Read on to understand how the Kotlin Primer is structured, what it contains and what it doesn't, and how it should be read.
What you won’t find in the Kotlin Primer
Subjects outside the JVM
The Kotlin Primer is focused solely on the JVM.
Coroutines
I strive to provide a certain level of quality to the readers of the Kotlin Primer. I'm not satisfied with explanations that involve hand waving, and I expect to be able to dissect every issue down to the fundamental level necessary to truly understand a given topic.
A direct prerequisite for this is a satisfactory amount of practical experience, not only with the topic, but also with learning the topic. If there is any quality to the Kotlin Primer, it is a consequence of having undertaken many paths through the material, from those that are readily available elsewhere, to those that are a result of experimentation, introspection, or challenges one puts oneself to, and then looking back and picking the one which is deemed most satisfactory.
I don’t yet feel that I have reached this threshold with coroutines, and I currently don’t feel confident the material would live up to these standards of quality.
One or two newer features
The bulk of the Kotlin Primer was written during the year 2021, based on experience gathered during previous years. Therefore, certain newer features that have since arrived are not covered in these materials, for reasons similar to those stated above. I will cover these subjects once I am satisfied with the degree to which I understand them.
Over-the-top political correctness
Though used sparingly, there are times when “fucking” and “bullshit” are the only words that truly capture the essence of a situation.
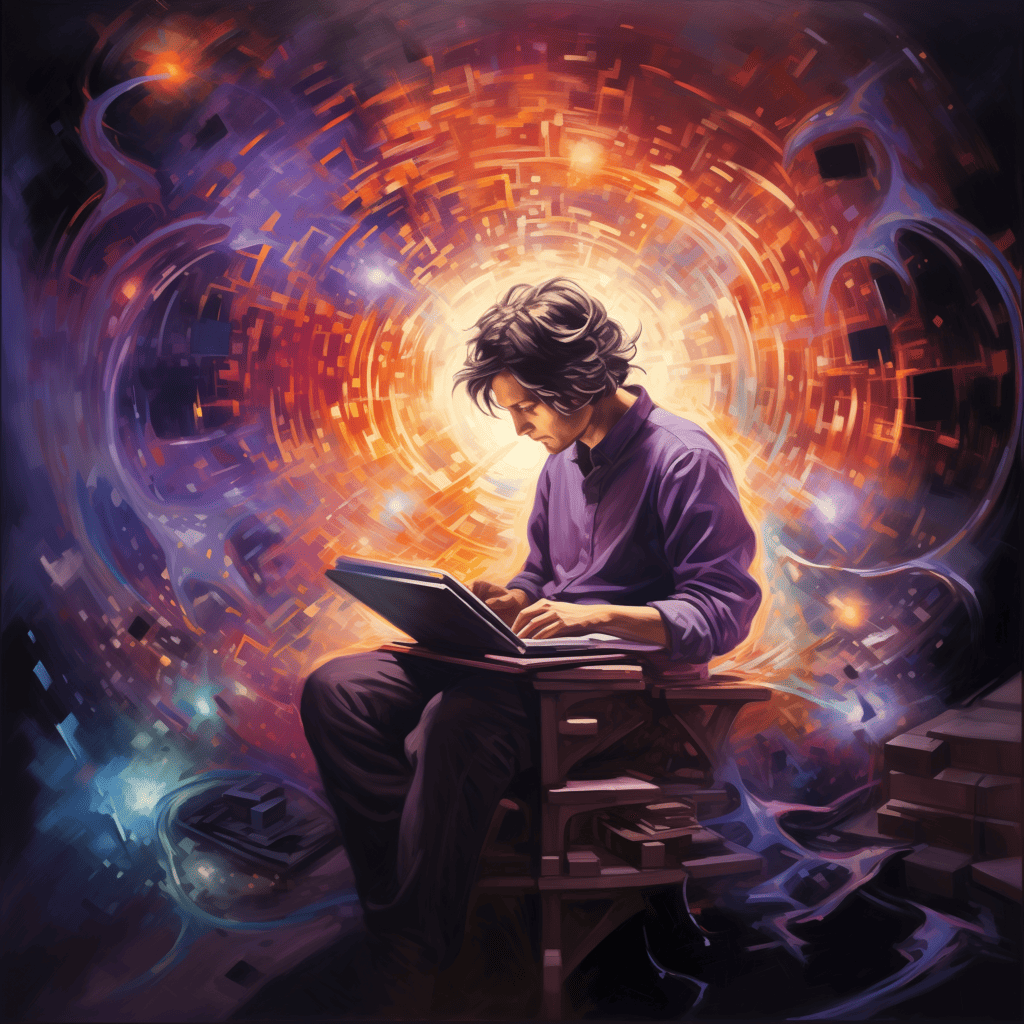
How to read the Kotlin Primer
Unless you are an avid reader, it is not necessary to read these materials from start to finish. You should instead browse through and concentrate on understanding two key things: 1) what features Kotlin offers, and 2) the problems they solve. Of these two, the latter is the most important — awareness of a problem will cause you to recognize it when it arises, and look for a solution. This will quickly lead you to the information you need.
I generally recommend against attempting to memorize all the details on the first go. Unless it’s something important, it’s usually better to just make a mental note that feature X is there for certain situations, and when you encounter these situations in real life, come back and read the section on feature X.
To help you decide what to concentrate on, each article in the Primer is tagged with the type of information included. These tags are:
🚙
- Mostly just FYI
- Often similar to what is available in Java, or not of large significance, and trivial to understand
- If you’ve never or only briefly used Kotlin, just quickly scroll through and be aware of what it is. No need to invest lots of time
- If you’ve already used Kotlin more than a few times, you can probably skip it
🚗
- Basically the same as 🚙, but also includes something extra that you might find interesting — a new concept, interpretation, link to interesting materials etc.
🏎️
- Describes a Kotlin feature
- Important and different from what’s available in Java, but shouldn’t be hard to understand
- If you’ve never or only briefly used Kotlin, read through carefully and make sure you understand the principles of the feature and when you should use it. Then come back and read it again when you first encounter a situation where you'd use it.
- If you’ve already used Kotlin more than a few times, just quickly scroll through and check if maybe there’s some aspect you didn’t know of
🚀
- Describes a fundamental concept
- Completely different from what’s available in Java and also may require a new style of thinking to understand and use effectively
- You should invest the time and read through this, regardless of your seniority level. There is a distinct possibility you will learn something new, be confronted with a novel interpretation, or discover motivation for using it in a particular manner
⚙️
- Contains exercises
- Exercises are included for most 🚀 and 🏎️, but not for the rest — I see no point in “Create a
Dog
class that..." nonsense. - When an exercise is there, it’s there for a reason — do it.
Book Sections
Introduction
- Describes basic language syntax and the type system
- Pay special attention to
when
, string templates, nullability and higher-order functions/lambdas. Understand that almost everything is an expression - I recommend reading the “expanded” version of types, but that can be left for later
- Make mental notes about ranges,
tailrec
,inline
andvararg
Classes — What we know from Java
- Lengthy section on the aspects of classes that differ only slightly from what is available in Java
- Mainly included as a reference
- Be sure to understand the fundamentals, as they are different from Java, especially the primary constructor and properties
- I recommend reading the section on variance — even if you understand the concepts, it might give you a different point of view
- The rest of the section on generics might also warrant closer attention
- Other than that, just sort of fly through quickly, there are almost no exercises
Classes — What is different
- Pay special attention to reified type parameters, data classes, sealed hierarchies, inline classes, objects and delegation — especially the last one can take time
- Dedicate time and energy to really understand the concept of modeling error states using sealed class hierarchies, and use it. It will make you a better programmer, and your code easier to maintain.
- Make mental notes of enums, infix functions, operators, type aliases and functional (SAM) interfaces
Extension functions
- Read the whole thing. No exceptions, everything is important
Standard library
- Pay special attention to Programming with Result — along with modeling errors states using classes, discussed in Classes — What is different, this is among the most important things you can learn from the Primer.
- Read the lesson on functional programming and why you should use it, why we needs Sequences, and pausing functions
- Other than that, almost no theory, just a demonstration of the stdlib
- Make mental note of everything
The Kotlin Playground
fun main() { cases("Hello") cases(1) cases(0L) cases(MyClass()) cases("hello") println(whenAssign("Hello")) println(whenAssign(3.4)) println(whenAssign(1)) println(whenAssign(MyClass())) } //sampleStart // Any in Kotlin is the same as Object in Java fun cases(obj: Any) { when (obj) { 1 -> println("One") "Hello" -> println("Greeting") is Long -> println("Long") !is String -> println("Not a string") else -> println("Unknown") } } class MyClass fun whenAssign(obj: Any): Any = when (obj) { 1 -> "one" "Hello" -> 1 is Long -> false else -> 42 } //sampleEnd
- Press the green play button in the top right corner to run the code.
- You can also edit the code if you want to.
- The (+) button in the center of the top edge expands the entire code.
- If the output of the code is long, it is sometimes better to use the “Open in Playground” link in the lower left and run it directly in the Playground.
- A
main()
function is necessary to run code examples (except for exercises), and one is not always provided. Feel free to add it yourself if you’re experimenting and need the code to output something.
Supported keyboard shortcuts
[Ctrl]+[Space]
— code completion[Ctrl]+[F9] / [⌘]+[R]
— execute snippet[Ctrl]+[/]
— comment code[Ctrl]+[Alt]+[L]
/[⌘]+[Alt]+[L]
— format code[Shift]+[Tab]
— decrease indent[Ctrl]+[Alt]+[H]
/[⌘]+[Alt]+[H]
— highlight code[Ctrl]+[Alt]+[Enter]
/[⌘]+[Alt]+[Enter]
— show import suggestions
Images
All images were generated with DALL·E 2 or Midjourney, unless otherwise specified in the caption.
Read on to discover the importance of maintainability, and how the Kotlin Primer emphasizes communicating intent and involving the compiler.
Tnx
You’re very welcome!